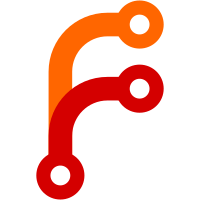
- Adds price tag calculation. Addons tagged #OPAQUE are excluded from the price tag. - BREAKING CHANGE: instead of abusing $product->{price} for a percent, $product->{percent} is no longer a boolean but the actual percent, so $product->{price} is the calculated amount. The total price of a product is now calculated in two places, once when reading the product list, and once as the result of adding the entry and its contras when adding the product. Although this involves some duplication and the sums are calculated in different ways, it hinges on the existing assertion to make sure that the entry is balanced to ensure that both sums are the same. Because of that, this code duplication actually strengthens the integrity.
53 lines
1.3 KiB
Perl
53 lines
1.3 KiB
Perl
#!perl
|
|
use RevBank::Products qw(read_products);
|
|
|
|
HELP1 "<productID>" => "Add a product to pending transaction";
|
|
|
|
sub command :Tab(&tab) ($self, $cart, $command, @) {
|
|
$command =~ /\S/ or return NEXT;
|
|
$command =~ /^\+/ and return NEXT;
|
|
|
|
my $products = read_products;
|
|
|
|
my $product = $products->{ $command } or return NEXT;
|
|
my $price = $product->{price};
|
|
|
|
my $entry = $cart->add(
|
|
-$product->{total_price},
|
|
$product->{description},
|
|
{
|
|
product_id => $product->{id},
|
|
plugin => $self->id,
|
|
product => $product,
|
|
deduplicate => join("/", $self->id, $product->{id}),
|
|
}
|
|
);
|
|
|
|
my $contra_desc = "\$you bought $product->{description}";
|
|
my @addons = @{ $product->{addons} // [] };
|
|
my $display = undef;
|
|
$display = "Product" if @addons and $price->cents > 0;
|
|
$display = "Reimbursement" if @addons and $price->cents < 0;
|
|
|
|
$entry->add_contra(
|
|
$product->{contra},
|
|
+$price,
|
|
$contra_desc,
|
|
$display
|
|
);
|
|
|
|
for my $addon (@addons) {
|
|
$entry->add_contra(
|
|
$addon->{contra},
|
|
$addon->{price},
|
|
"$addon->{description} ($contra_desc)",
|
|
$addon->{description}
|
|
);
|
|
}
|
|
|
|
return ACCEPT;
|
|
}
|
|
|
|
sub tab {
|
|
return grep !/^\+/, grep /\D/, keys %{ read_products() };
|
|
}
|