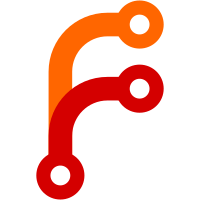
(Bumps version to 3.8 because admins should update the plugin list.) Deduplication didn't work on quantified additions, i.e. if you added "20x clubmate" when there was already clubmate in the cart, it would add just ONE item, and have a lingering message that the next thing would be multiplied by 20. This old bug was especially annoying if there is a barcode "20x clubmate" to scan 20 bottles (which is the size of a crate), and this is repeated. The fix also uncovered another bug: newly added entries were selected too early. There are two hooks, hook_add_entry and hook_added_entry, and of course the selection should happen in between, not before the former. No entry in UPGRADING.md, because I think it is extremely unlikely that any plugin author will have used the selection feature yet, which is very new.
61 lines
1.5 KiB
Perl
61 lines
1.5 KiB
Perl
#!perl
|
|
|
|
HELP "market" => "Edit market list";
|
|
|
|
my $filename = 'revbank.market';
|
|
|
|
sub _read_market() {
|
|
my %market;
|
|
for (slurp $filename) {
|
|
/^\s*#/ and next;
|
|
/\S/ or next;
|
|
chomp;
|
|
my ($user, $id, $seller, $space, $description) = split " ", $_, 5;
|
|
$market{$id} = {
|
|
user => $user,
|
|
seller => $seller,
|
|
space => $space,
|
|
description => $description,
|
|
};
|
|
}
|
|
return \%market;
|
|
}
|
|
|
|
sub command :Tab(market,&tab) ($self, $cart, $command, @) {
|
|
if ($command eq 'market') {
|
|
require RevBank::TextEditor;
|
|
RevBank::TextEditor::edit($filename);
|
|
return ACCEPT;
|
|
}
|
|
|
|
my $product = _read_market->{ $command } or return NEXT;
|
|
|
|
my $username = parse_user( $product->{ user }) or return NEXT;
|
|
my $seller = parse_amount($product->{ seller }) or return NEXT;
|
|
my $space = parse_amount($product->{ space }) or return NEXT;
|
|
my $description = $product->{description};
|
|
|
|
$cart->add(
|
|
-($seller + $space),
|
|
"$description (sold by $username)",
|
|
{
|
|
product_id => $command,
|
|
plugin => $self->id,
|
|
deduplicate => join("/", $self->id, $command),
|
|
}
|
|
)->add_contra(
|
|
$username,
|
|
$seller,
|
|
"\$you bought $description"
|
|
)->add_contra(
|
|
"+sales/market",
|
|
$space,
|
|
"\$you bought $description from $username"
|
|
);
|
|
|
|
return ACCEPT;
|
|
}
|
|
|
|
sub tab {
|
|
return grep /\D/, keys %{ _read_market() };
|
|
}
|